Touchpoint Guides
Changing Chat Modes
Touchpoint UI offers two distinct chat interface modes to suit different user experience needs.
Chat Modes
Mode | Description | Best Used When | Key Benefits |
---|---|---|---|
Assistant Style | Focused interface highlighting current interaction | Guided tasks, step-by-step workflows, single interactions | Clean minimal interface, focused attention, reduced distraction |
Classic Chat Style | Traditional messaging interface with history | Complex discussions, reference-heavy interactions | Full history visible, easy reference, familiar experience |
Assistant Style (Default)
The Assistant Style provides a focused experience where only the latest application message is prominently displayed. This creates a clean interface that directs the user's attention to the most recent information or question, while still allowing them to scroll up to view conversation history.
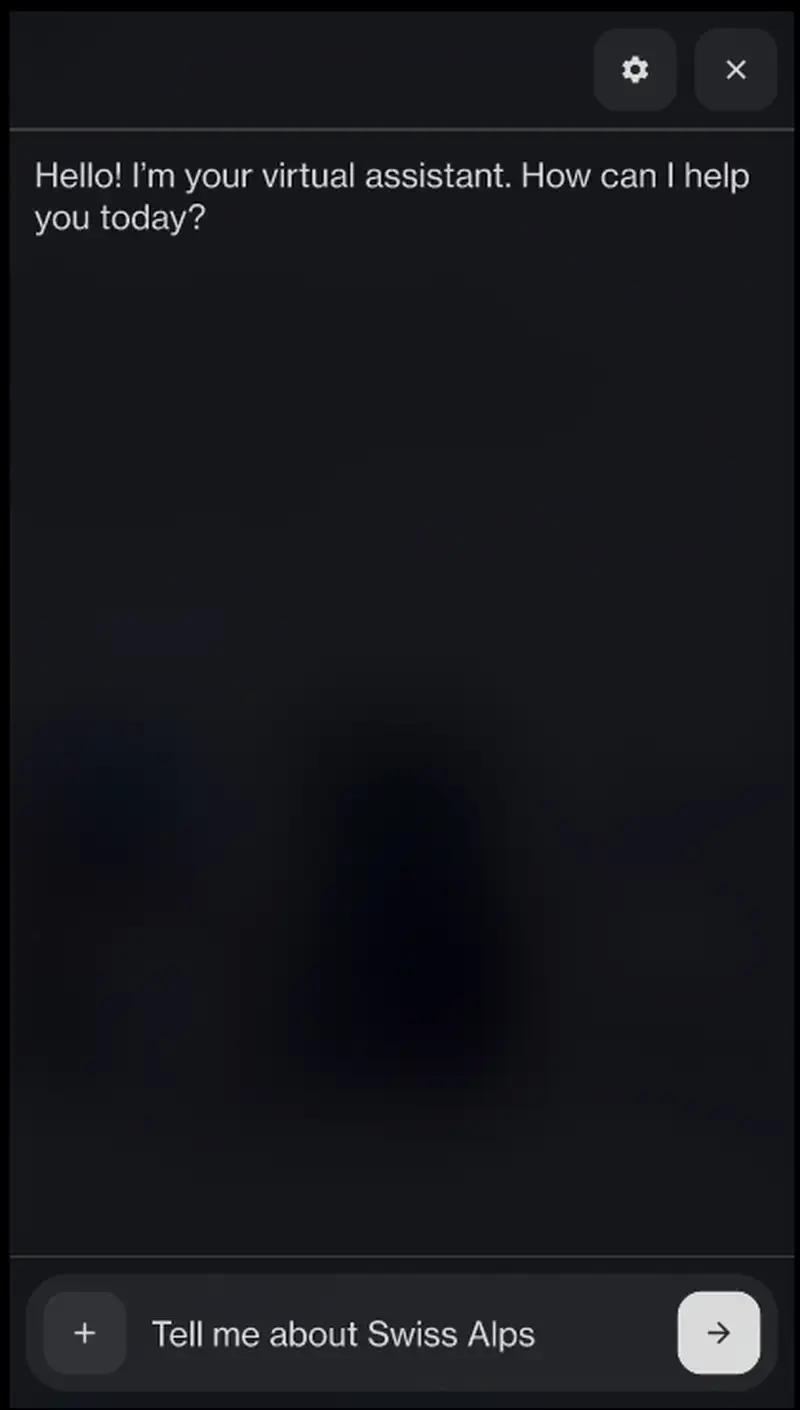
Example Assistant Configuration
To implement the default Assistant Style, you can either omit the chatMode
parameter or explicitly set it to false
:
1import { create } from "@nlxai/touchpoint-ui"; 2 3const touchpointOptions = { 4 config: { 5 applicationUrl: "YOUR_APPLICATION_URL", 6 headers: { 7 "nlx-api-key": "YOUR_API_KEY", 8 }, 9 languageCode: "en-US", 10 }, 11 chatMode: false, // Explicitly set to false or omit (default is false) 12 // Add other common options like theme, brandIcon etc. if needed 13 theme: { 14 fontFamily: '"Neue Haas Grotesk", sans-serif', 15 accent: "#AECAFF", 16 }, 17}; 18 19const touchpoint = await create(touchpointOptions);
Example Assistant Configuration with message bubbles
To add message bubbles to the Assistant Style:
1import { create } from "@nlxai/touchpoint-ui"; 2 3const touchpointOptions = { 4 config: { 5 applicationUrl: "YOUR_APPLICATION_URL", 6 headers: { 7 "nlx-api-key": "YOUR_API_KEY", 8 }, 9 languageCode: "en-US", 10 }, 11 chatMode: false, // Assistant style 12 userMessageBubble: true, // Display user messages in bubbles 13 agentMessageBubble: true, // Display agent messages in bubbles 14 theme: { 15 fontFamily: '"Neue Haas Grotesk", sans-serif', 16 accent: "#AECAFF", 17 }, 18}; 19 20const touchpoint = await create(touchpointOptions);
Classic Chat Style
The Classic Chat Style provides a traditional messaging interface where all messages stack chronologically and remain visible. This creates a more conventional chat experience that many users are already familiar with from messaging apps.
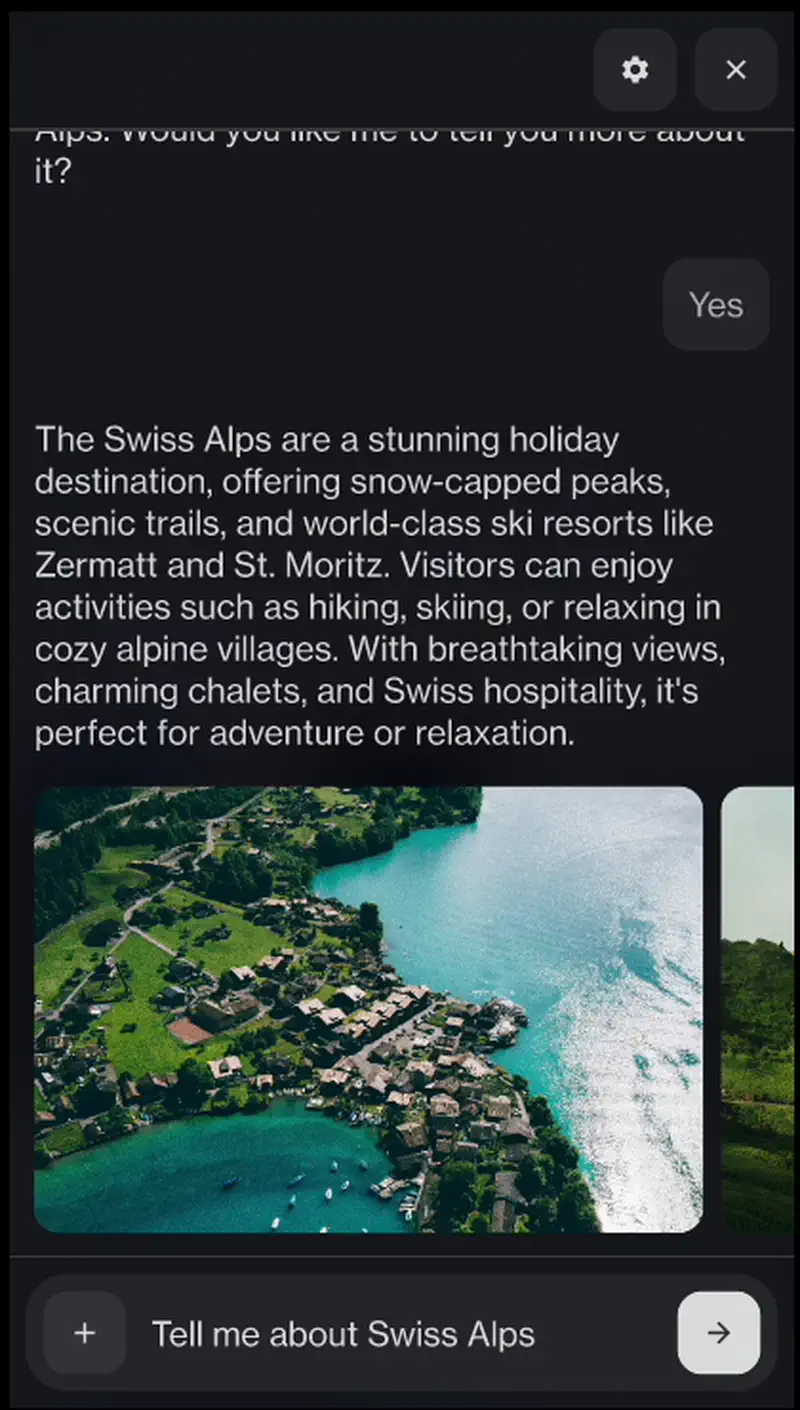
Example Classic Chat Configuration
To implement the Classic Chat Style, set the chatMode
parameter to true
:
1import { create } from "@nlxai/touchpoint-ui"; 2 3const touchpointOptions = { 4 config: { 5 applicationUrl: "YOUR_APPLICATION_URL", 6 headers: { 7 "nlx-api-key": "YOUR_API_KEY", 8 }, 9 languageCode: "en-US", 10 }, 11 chatMode: true, // Enable classic chat mode 12 // Add other common options like theme, brandIcon etc. if needed 13 theme: { 14 fontFamily: '"Arial", sans-serif', 15 accent: "rgb(40, 167, 69)", // Example green accent 16 }, 17}; 18 19const touchpoint = await create(touchpointOptions);
Example Classic Chat Configuration with message bubbles
To enable message bubbles in Classic Chat Style for a more traditional messaging look:
1import { create } from "@nlxai/touchpoint-ui"; 2 3const touchpointOptions = { 4 config: { 5 applicationUrl: "YOUR_APPLICATION_URL", 6 headers: { 7 "nlx-api-key": "YOUR_API_KEY", 8 }, 9 languageCode: "en-US", 10 }, 11 chatMode: true, // Enable classic chat mode 12 userMessageBubble: true, // Display user messages in bubbles 13 agentMessageBubble: true, // Display agent messages in bubbles 14 theme: { 15 fontFamily: '"Arial", sans-serif', 16 accent: "rgb(40, 167, 69)", // Example green accent 17 }, 18}; 19 20const touchpoint = await create(touchpointOptions);
For more information on customizing your Touchpoint UI experience, refer to the Theming documentation and Component Configuration guides.