Touchpoint components
Buttons
Text Button Example
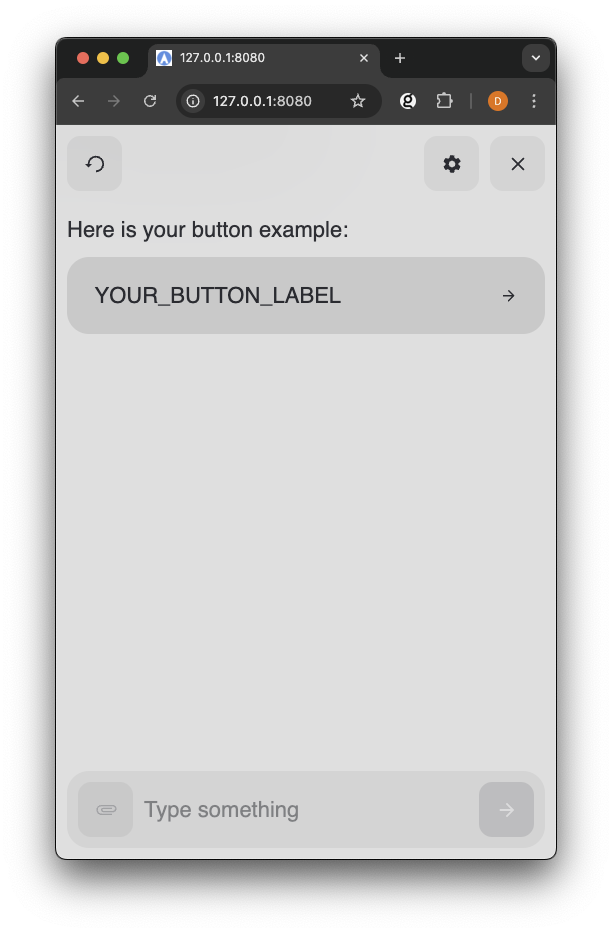
About
Touchpoint provides two types of button components for handling user interactions: TextButton and IconButton. These components offer consistent styling and behavior while serving different interaction needs.
- TextButton - Text-based interactive element with optional icon support. It's ideal for primary actions and menu items.
- IconButton - Icon-only interactive element. It's ideal for compact actions and toolbars.
Properties
Both TextButton and IconButton have required properties to render the elements correctly in the conversations flow.
TextButton Properties
Property | Type | Required | Description |
---|---|---|---|
label | string | Yes | Button text |
Icon | Icon | Yes | Icon component |
onClick | function | No | Click handler |
type |
| No | Button style variant |
IconButton Properties
Property | Type | Required | Description |
---|---|---|---|
Icon | Icon | Yes | Icon component to render |
label | string | Yes | Accessible label (for screen readers) |
type |
| Yes | Button style variant |
onClick | function | No | Click handler |
Import and Basic Usage
You can import the buttons elements from touchpoint once the package has been installed or made available in your project.
Define onClick
The Button Component expect a function passed via onClick
to define the actions to take when a user clicks the button.
Access the ConversationHandler method sendChoice
via conversationHandler.sendChoice
to send the user's choice back to NLX.
Read more details about building Custom Components with Touchpoint in the Getting started with Touchpoint components documentation page.
Example
The examples below require a modality defined in your NLX application as an object with at least buttonLabel
and buttonId
properties.
Example Modality Schema
1{ 2 "buttonLabel": "Label passed to button Component", 3 "buttonId": "Id of the 'choice' to send back to NLX" 4}
Example Button Components
1import { TextButton, IconButton, Icons } from "@nlxai/touchpoint-ui"; 2 3const TextButtonExample = ({ data, conversationHandler }) => { 4 return ( 5 <TextButton 6 label={data.buttonLabel} 7 Icon={Icons.ArrowForward} 8 onClick={() => conversationHandler.sendChoice(data.buttonId)} 9 /> 10 ); 11}; 12 13const IconButtonExample = ({ data, conversationHandler }) => { 14 return ( 15 <IconButton 16 label={data.buttonLabel} 17 Icon={Icons.ArrowForward} 18 onClick={() => conversationHandler.sendChoice(data.buttonId)} 19 type="main" 20 /> 21 ); 22};
Related Documents
- Icons for list of Icons available in Touchpoint
- Theming Touchpoint
- Building Components without JSX